RTC Module(DS1302)
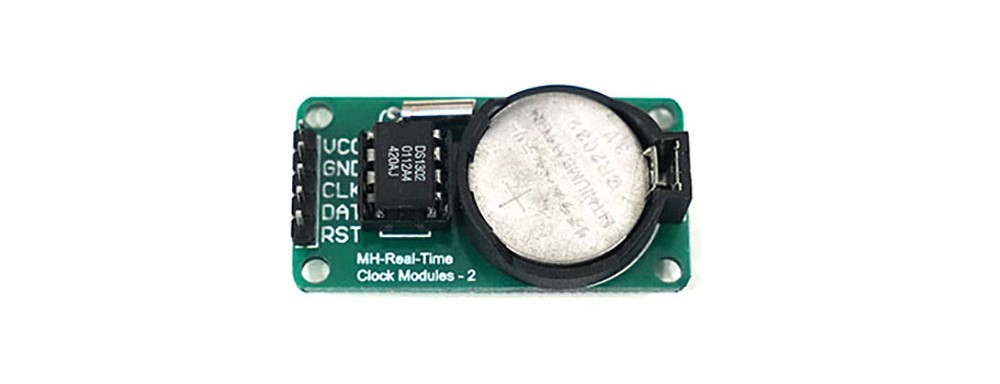
This is a module that can remember the time even when it is not connected to a board.
It uses a battery (CR2032).
Specifications
- Operating Voltage: 3.3V ~ 5V
- Operating Current: 300nA at 2V
Required Hardware
- RTC Module
- Arduino
Connections
RTC module | Arduino |
VCC | 5V |
GND | GND |
CLK | D5 |
DAT | D4 |
RST | D3 |
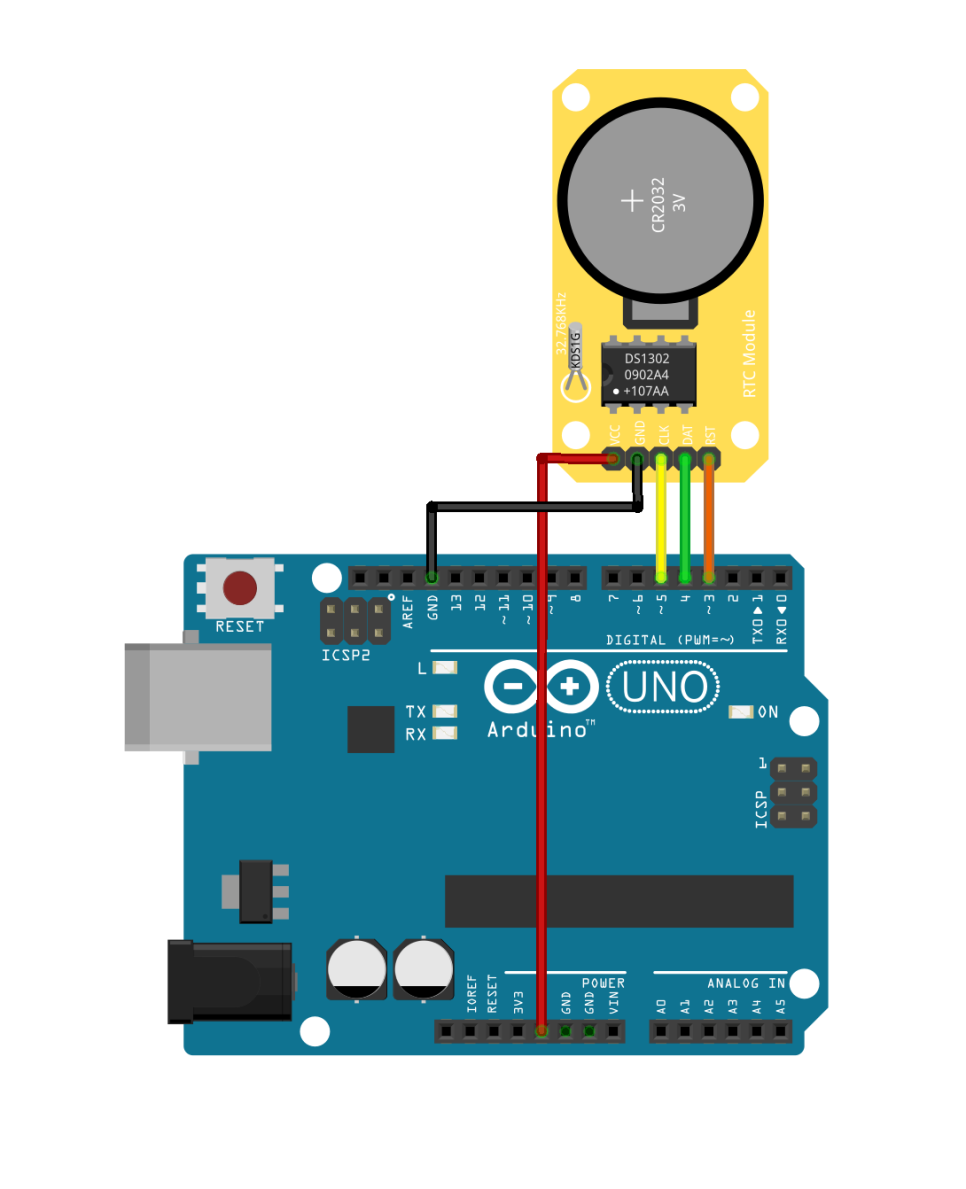
Library
This document uses the Rtc by Makuna library for Arduino. Arduino Libraries
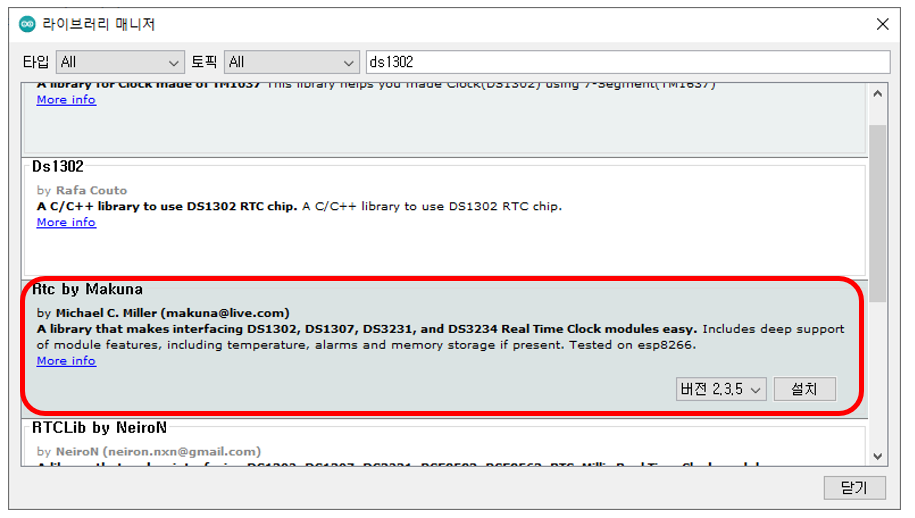
Example Code
The code stores the time at the moment of compilation. There will be a slight difference when uploaded to the board due to the time taken for the upload (since the board is uploaded after compiling, there is a time discrepancy).
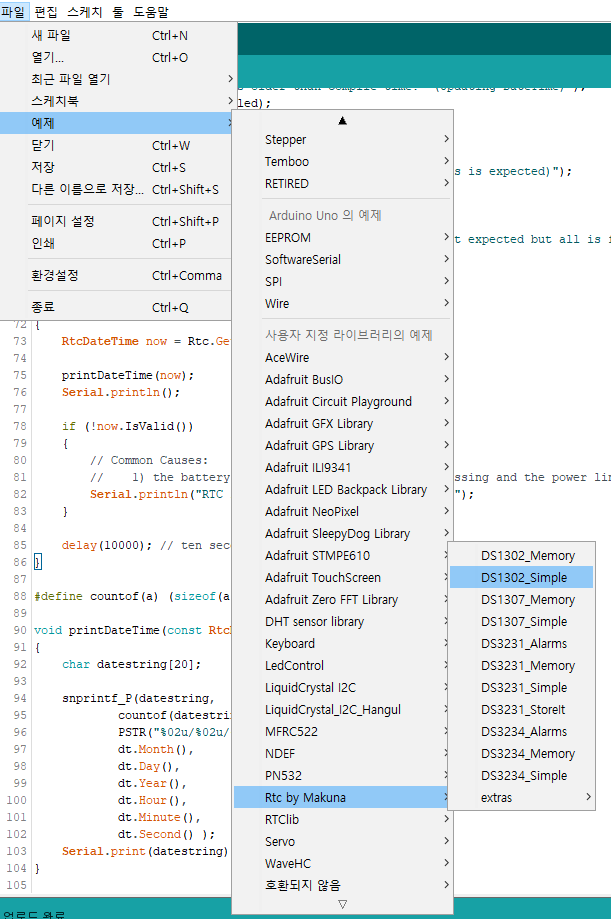
#include <ThreeWire.h>
#include <RtcDS1302.h>
//--------------------Modified Section-------------------------------
#define CLK 5 //Specify CLK pin number
#define DAT 4 //Specify DAT pin number
#define RST 3 //Specify RST pin number
ThreeWire myWire(DAT,CLK,RST);
//--------------------------------------------------------------
RtcDS1302<ThreeWire> Rtc(myWire);
void setup ()
{
Serial.begin(57600);
Serial.print("compiled: ");
Serial.print(__DATE__);
Serial.println(__TIME__);
Rtc.Begin();
RtcDateTime compiled = RtcDateTime(__DATE__, __TIME__);
printDateTime(compiled);
Serial.println();
if (!Rtc.IsDateTimeValid())
{
// Common Causes:
// 1) first time you ran and the device wasn't running yet
// 2) the battery on the device is low or even missing
Serial.println("RTC lost confidence in the DateTime!");
Rtc.SetDateTime(compiled);
}
if (Rtc.GetIsWriteProtected())
{
Serial.println("RTC was write protected, enabling writing now");
Rtc.SetIsWriteProtected(false);
}
if (!Rtc.GetIsRunning())
{
Serial.println("RTC was not actively running, starting now");
Rtc.SetIsRunning(true);
}
RtcDateTime now = Rtc.GetDateTime();
if (now < compiled)
{
Serial.println("RTC is older than compile time! (Updating DateTime)");
Rtc.SetDateTime(compiled);
}
else if (now > compiled)
{
Serial.println("RTC is newer than compile time. (this is expected)");
}
else if (now == compiled)
{
Serial.println("RTC is the same as compile time! (not expected but all is fine)");
}
}
void loop ()
{
RtcDateTime now = Rtc.GetDateTime();
printDateTime(now);
Serial.println();
if (!now.IsValid())
{
// Common Causes:
// 1) the battery on the device is low or even missing and the power line was disconnected
Serial.println("RTC lost confidence in the DateTime!");
}
delay(10000); // ten seconds
}
#define countof(a) (sizeof(a) / sizeof(a[0]))
void printDateTime(const RtcDateTime& dt)
{
char datestring[20];
snprintf_P(datestring,
countof(datestring),
PSTR("%02u/%02u/%04u %02u:%02u:%02u"),
dt.Month(),
dt.Day(),
dt.Year(),
dt.Hour(),
dt.Minute(),
dt.Second() );
Serial.print(datestring);
}
Execution Result
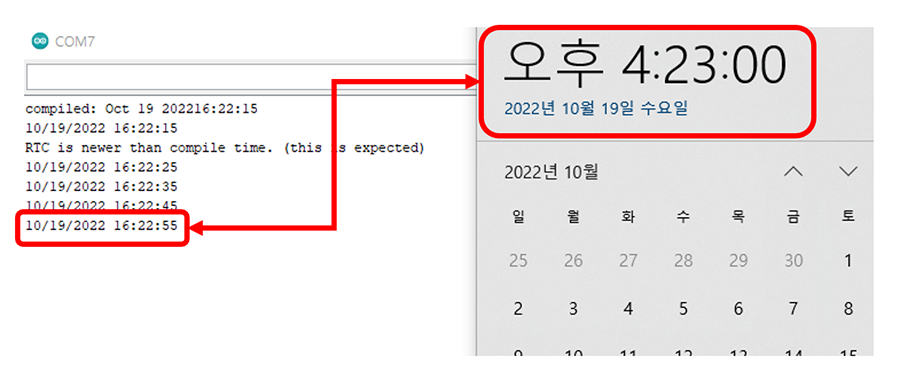