RTC 모듈(DS1302)
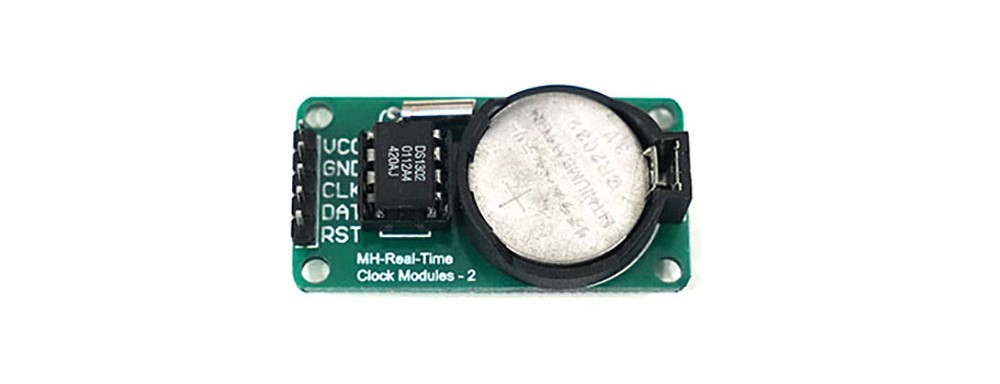
보드와 연결되어 있지 않아도 시간을 기억하는 모듈입니다.
배터리(CR2032)를 사용합니다.
사양
- 작동전압 : 3.3V ~ 5V
- 작동전류 : 2V 기준 300nA
필요 하드웨어
- RTC 모듈
- Arduino
연결
RTC module | Arduino |
VCC | 5V |
GND | GND |
CLK | D5 |
DAT | D4 |
RST | D3 |
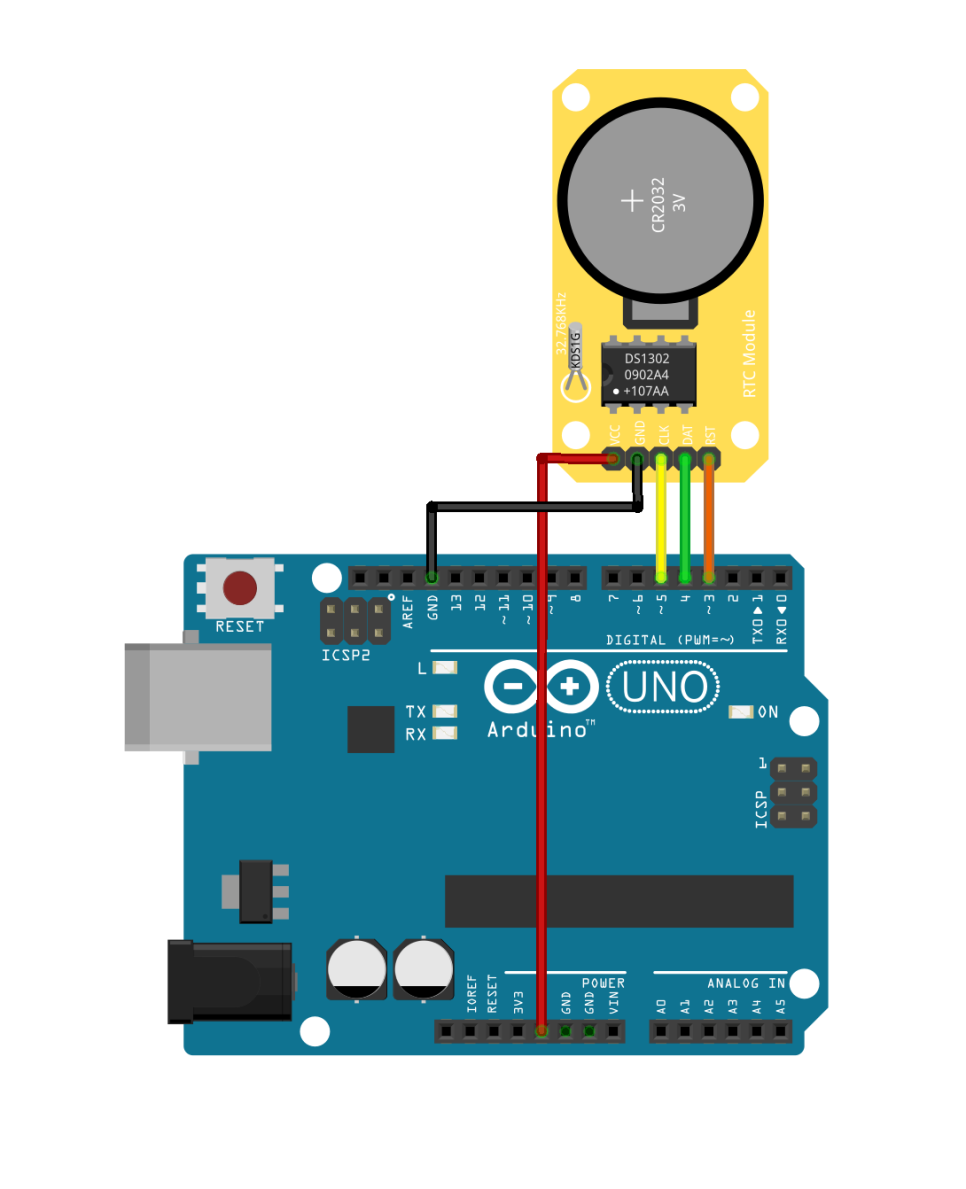
라이브러리
본문에서는 Rtc by Makuna 라이브러리를 사용합니다. 아두이노 라이브러리
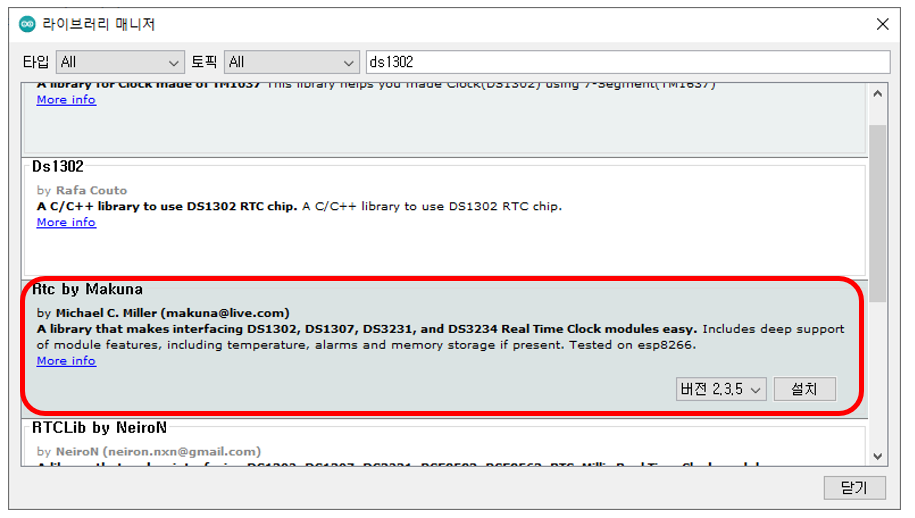
예제 코드
컴파일 시점에서 시간을 저장하는 코드로 보드에 업로드 시 약간의 차이가 발생합니다.
(코드 컴파일 후 보드에 업로딩 되므로 업로드되는 시간동안 오차 발생)
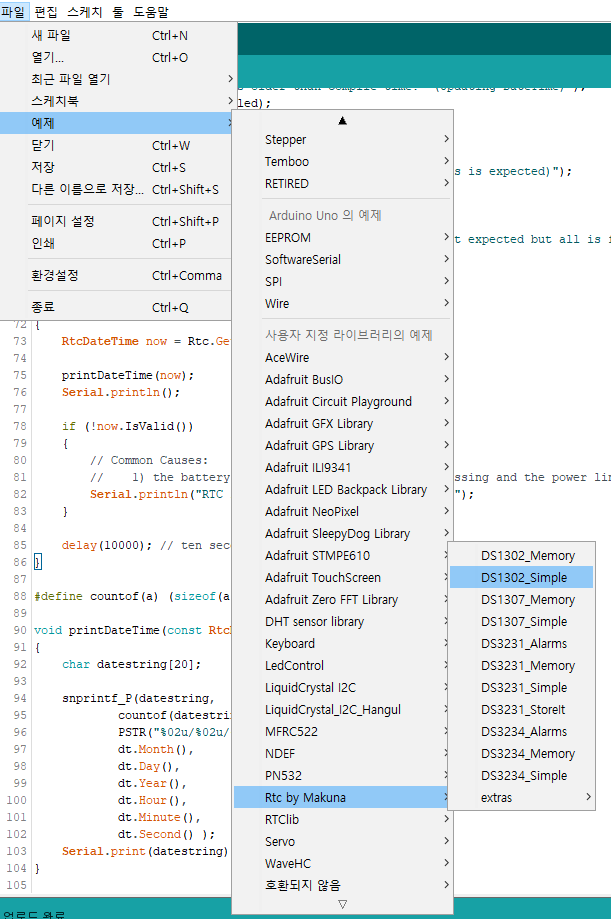
#include <ThreeWire.h>
#include <RtcDS1302.h>
//--------------------수정한 부분--------------------------------
#define CLK 5 //CLK 핀번호 지정
#define DAT 4 //DAT 핀번호 지정
#define RST 3 //RST 핀번호 지정
ThreeWire myWire(DAT,CLK,RST);
//--------------------------------------------------------------
RtcDS1302<ThreeWire> Rtc(myWire);
void setup ()
{
Serial.begin(57600);
Serial.print("compiled: ");
Serial.print(__DATE__);
Serial.println(__TIME__);
Rtc.Begin();
RtcDateTime compiled = RtcDateTime(__DATE__, __TIME__);
printDateTime(compiled);
Serial.println();
if (!Rtc.IsDateTimeValid())
{
// Common Causes:
// 1) first time you ran and the device wasn't running yet
// 2) the battery on the device is low or even missing
Serial.println("RTC lost confidence in the DateTime!");
Rtc.SetDateTime(compiled);
}
if (Rtc.GetIsWriteProtected())
{
Serial.println("RTC was write protected, enabling writing now");
Rtc.SetIsWriteProtected(false);
}
if (!Rtc.GetIsRunning())
{
Serial.println("RTC was not actively running, starting now");
Rtc.SetIsRunning(true);
}
RtcDateTime now = Rtc.GetDateTime();
if (now < compiled)
{
Serial.println("RTC is older than compile time! (Updating DateTime)");
Rtc.SetDateTime(compiled);
}
else if (now > compiled)
{
Serial.println("RTC is newer than compile time. (this is expected)");
}
else if (now == compiled)
{
Serial.println("RTC is the same as compile time! (not expected but all is fine)");
}
}
void loop ()
{
RtcDateTime now = Rtc.GetDateTime();
printDateTime(now);
Serial.println();
if (!now.IsValid())
{
// Common Causes:
// 1) the battery on the device is low or even missing and the power line was disconnected
Serial.println("RTC lost confidence in the DateTime!");
}
delay(10000); // ten seconds
}
#define countof(a) (sizeof(a) / sizeof(a[0]))
void printDateTime(const RtcDateTime& dt)
{
char datestring[20];
snprintf_P(datestring,
countof(datestring),
PSTR("%02u/%02u/%04u %02u:%02u:%02u"),
dt.Month(),
dt.Day(),
dt.Year(),
dt.Hour(),
dt.Minute(),
dt.Second() );
Serial.print(datestring);
}
실행 결과
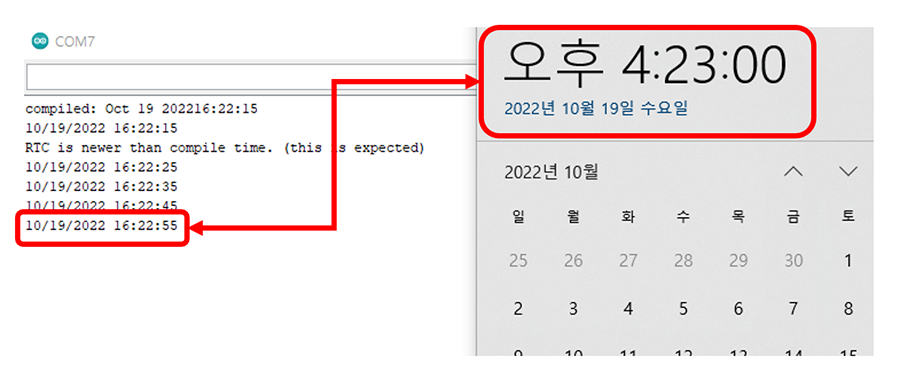