NeoPixel(WS2812)
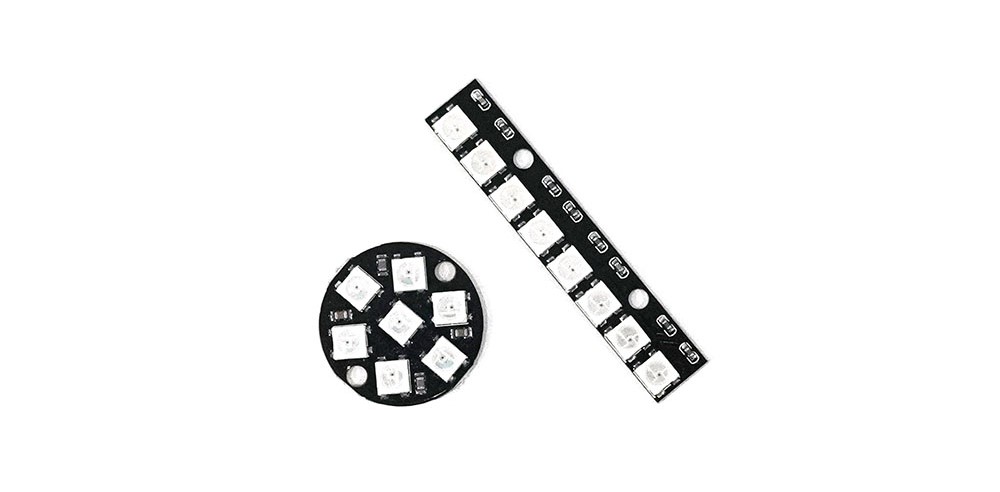
A single LED that can display multiple colors by adjusting RGB values, with each LED individually controllable by a controller.
In the text, two models were used: a circular one with 7 LEDs and a linear one with 8 LEDs.
The terminals total either 6 or 8, with the 6-terminal version comprising (IN, 2x VCC, 2x GND, OUT), and the 8-terminal version adding 2 more GNDs to the 6-terminal setup.
Connecting the OUT terminal of a NeoPixel to the IN terminal of the next allows for controlling multiple NeoPixels with just one pin on the board.
Specifications
- Operating Voltage: 5V
- Current per LED: 20mA ~ 80mA
Required Hardware
- NeoPixel
- Arduino UNO
- UNO cable
- M-M cable(6ea)
- F-M cable(3ea)
Connection (M-M and F-M cables were soldered for connection to the NeoPixel)
- NeoPixel to Arduino Connection
NeoPixel | Arduino |
---|---|
IN | D7 |
VCC | 5V |
GND | GND |
- Connection between the 1st and 2nd NeoPixel (apply the same method to add more NeoPixels):
1st NeoPixel | 2nd NeoPixel |
---|---|
OUT | IN |
VCC | VCC |
GND | GND |
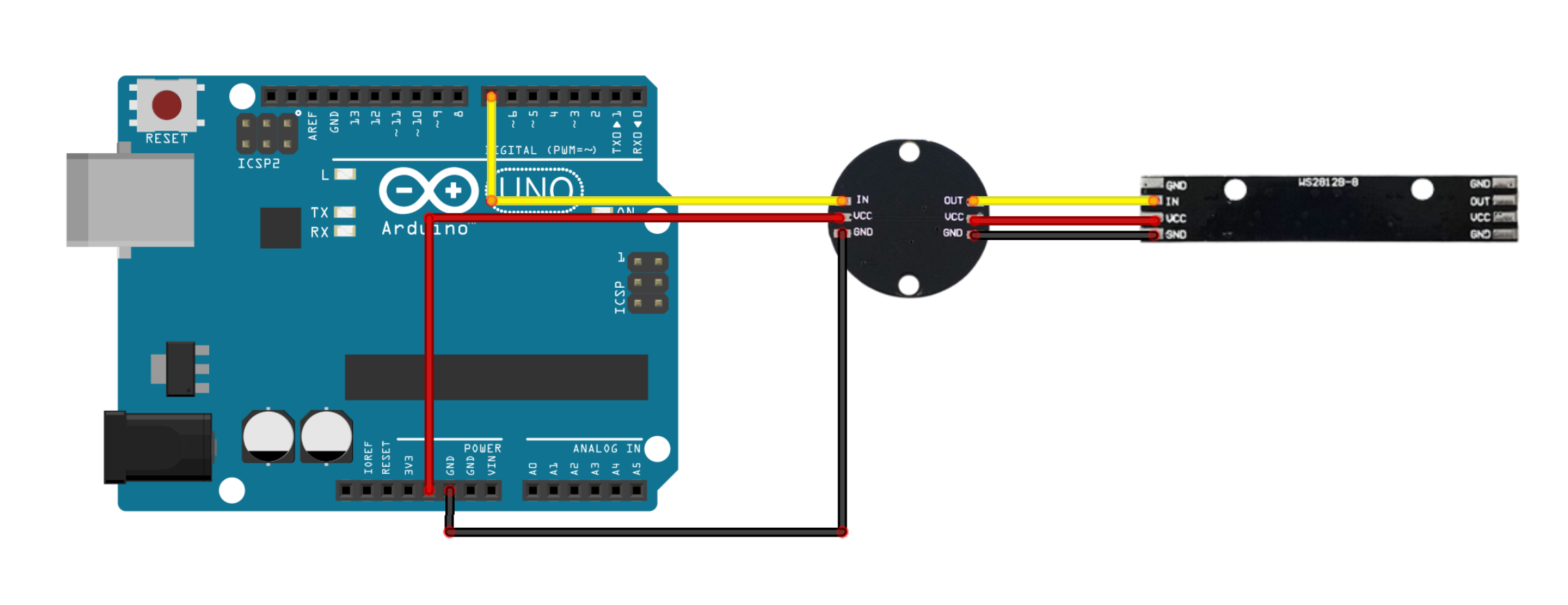
Library
- Adafruit NeoPixel (Arduino Libraries)
예제 코드
#include <Adafruit_NeoPixel.h>
// Using pin number 7
#define PIN 7
// Number of pixels
#define NUMPIXELS 15
// Brightness level
#define bright 255
#define dly 10000
// Create a NeoPixel object for control
Adafruit_NeoPixel neo(NUMPIXELS, PIN, NEO_GRB + NEO_KHZ800);
void setup() {
// Start NeoPixel
neo.begin();
// Set NeoPixel brightness
neo.setBrightness(bright);
// Initialize NeoPixel
neo.clear();
// Apply NeoPixel settings
// Settings are applied internally until .show() is executed.
// When .show() runs, the commands applied internally are executed on the LEDs.
neo.show();
}
void loop()
{
// Turn LEDs white
for (int i = 0; i < 15; i++)
{
neo.setPixelColor(i, 255, 255, 255);
}
neo.show();
delay(500);
// Turn LEDs red
for (int i = 0; i < 15; i++)
{
neo.setPixelColor(i, 255, 0, 0);
}
neo.show();
delay(500);
// Turn LEDs green
for (int i = 0; i < 15; i++)
{
neo.setPixelColor(i, 0, 255, 0);
}
neo.show();
delay(500);
// Turn LEDs blue
for (int i = 0; i < 15; i++)
{
neo.setPixelColor(i, 0, 0, 255);
}
neo.show();
delay(500);
}
Execution Result
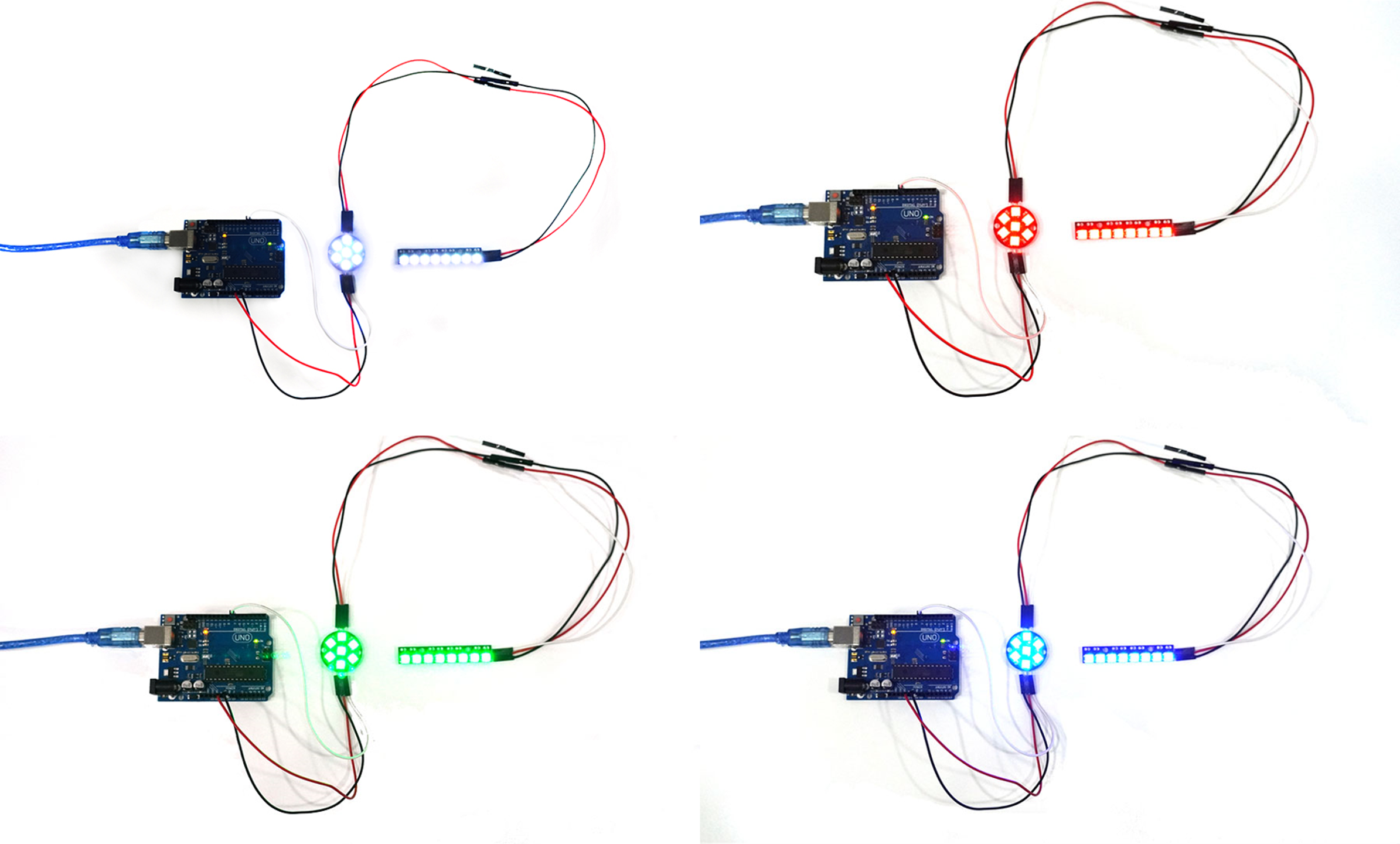