Neopixel Ring 24-LED(WS2812)
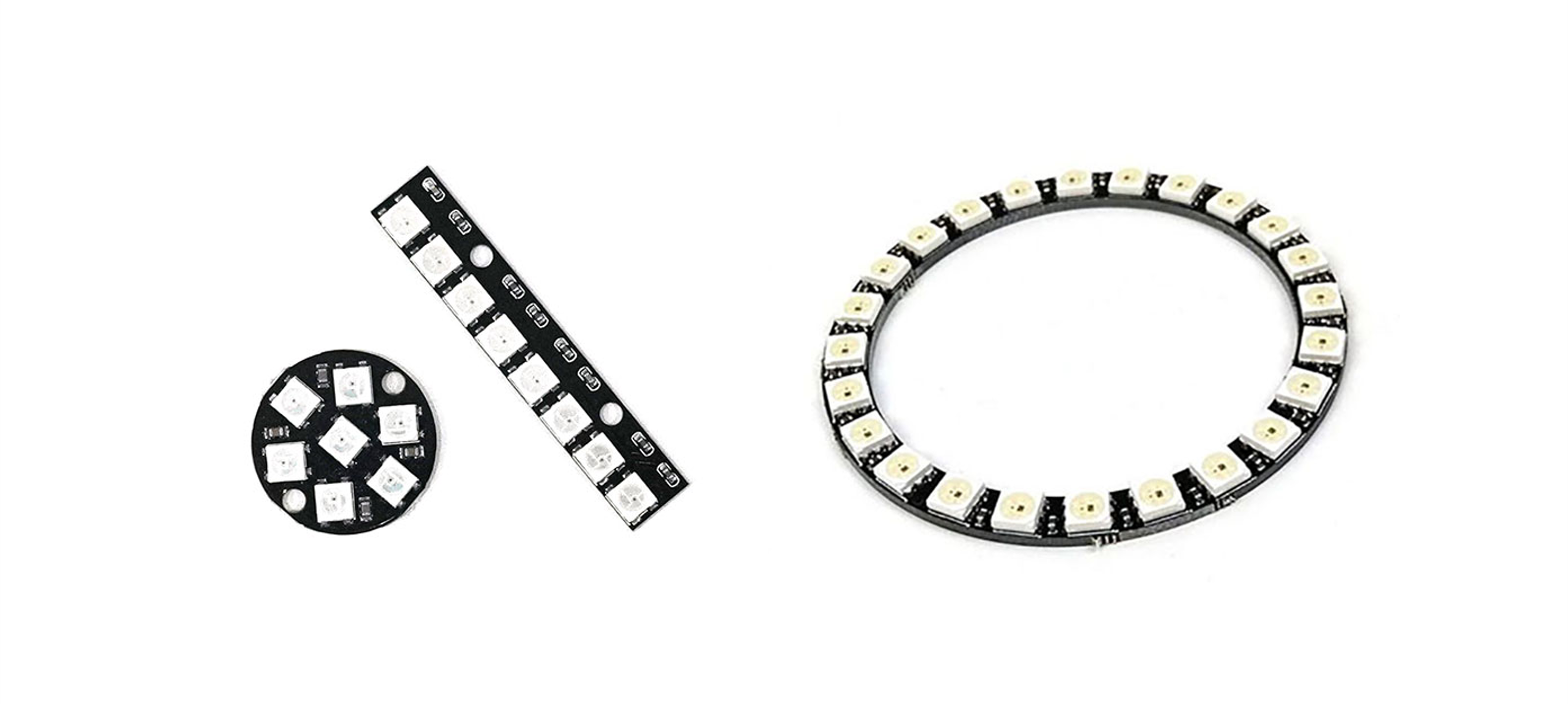
Neopixel is an LED product from Adafruit that has a WS281x chip built-in.
It comes in various shapes like single, circular, and linear, making it convenient for different applications, with the advantage of easy wiring.
It is commonly used with microcontrollers like Arduino.
Specifications
- Operating Voltage: 5V
- Current per LED: 20mA to 80mA
Example Hardware
- Arduino Board
- 24-bit Neopixel
Connection
This component does not come with pre-attached wires, so you need to solder jumper wires before proceeding.
Arduino | neo pixel |
5V | 5V |
GND | GND |
D7 | DI |
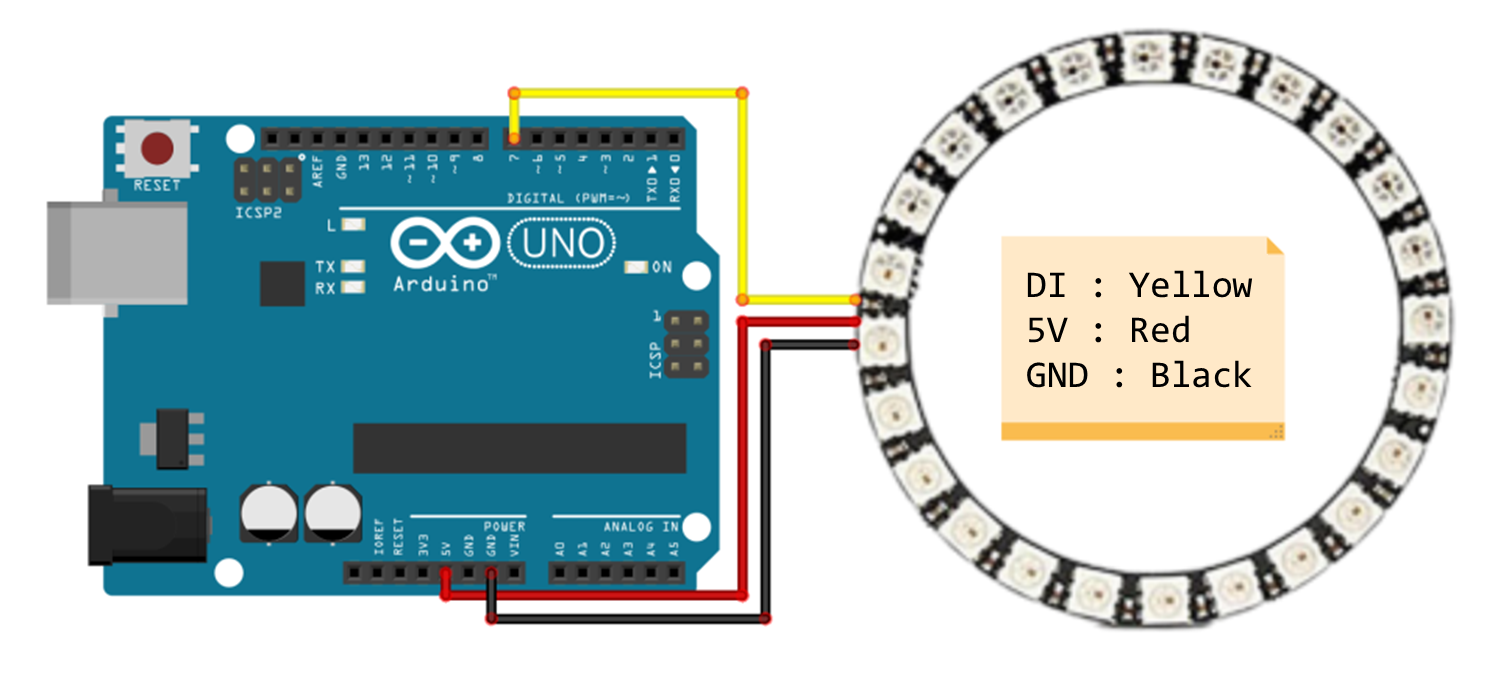
Library
Adafruit Neopixel library is used.
Please refer to the Arduino library documentation for how to use the library.
Example Code
This example sequentially lights up 7 colors.
#include <Adafruit_NeoPixel.h>
#define PIN 7
#define NUMPIXELS 24
#define bright 255
#define dly 50
Adafruit_NeoPixel neo(NUMPIXELS, PIN, NEO_GRB + NEO_KHZ800);
void setup() {
neo.begin();
neo.setBrightness(bright);
neo.clear();
neo.show();
for (int i = 0; i < NUMPIXELS; i++) {
neo.setPixelColor(i, 255, 255, 255);
neo.show();
delay(dly);
}
for (int i = 0; i < NUMPIXELS; i++) {
neo.setPixelColor(i, 255, 0, 0);
neo.show();
delay(dly);
}
for (int i = 0; i < NUMPIXELS; i++) {
neo.setPixelColor(i, 0, 255, 0);
neo.show();
delay(dly);
}
for (int i = 0; i < NUMPIXELS; i++) {
neo.setPixelColor(i, 0, 0, 255);
neo.show();
delay(dly);
}
for (int i = 0; i < NUMPIXELS; i++) {
neo.setPixelColor(i, 255, 255, 0);
neo.show();
delay(dly);
}
for (int i = 0; i < NUMPIXELS; i++) {
neo.setPixelColor(i, 0, 255, 255);
neo.show();
delay(dly);
}
for (int i = 0; i < NUMPIXELS; i++) {
neo.setPixelColor(i, 255, 0, 255);
neo.show();
delay(dly);
}
for (int i = 0; i < NUMPIXELS; i++) {
neo.setPixelColor(i, 0, 0, 0);
}
neo.show();
}
void loop() {
}
Execution Result
Please check the operational video from the link.