Ultrasonic Sensor(HC-SR04)
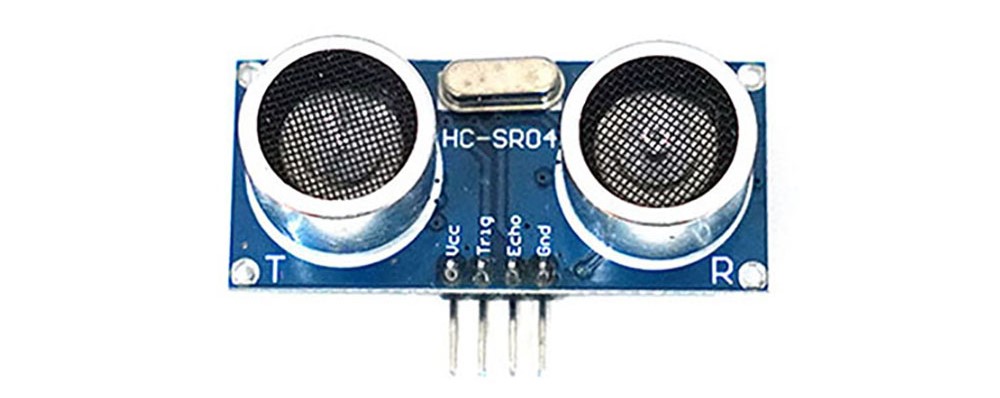
This is a sensor that measures distance using ultrasonic waves. The accuracy may vary depending on the environment. The process of measuring the distance value goes as follows:
[Ultrasonic emission -> Reflection of ultrasonic waves -> Detection of the returning ultrasonic waves].
Sensor Operating Principle
- Ultrasonic waves are generated through the trig pin.
- Immediately after the ultrasonic emission stops, the echo pin maintains a HIGH state.
- When the emitted ultrasonic waves return and are detected, the echo pin is changed to LOW.
- The time it takes for the ultrasonic waves to travel and return is obtained using pulseIn(echoPin, HIGH);.
- This time is then used in a formula to calculate the distance.
Measuring Distance
Ultrasonic Speed | 340m/s |
Round-trip time of ultrasonic waves | x |
Distance to the object | y |
Specifications
- Operating Voltage: 5V
- Operating Current: 15mA
- Operating Frequency: 40Hz
- Ultrasonic Frequency: 40kHz
- Maximum Detection Distance: 4m
- Minimum Detection Distance: 2cm
- Measurement Angle Range: 15 degrees
- Trigger Input: 10µs TTL pulse
Required Hardware
- Ultrasonic Sensor (HC-SR04)
- Arduino Board
- Jumper Cables
Connection
Trig and Echo can be connected to any desired pin number (in this document, pins D3 and D4 are used).
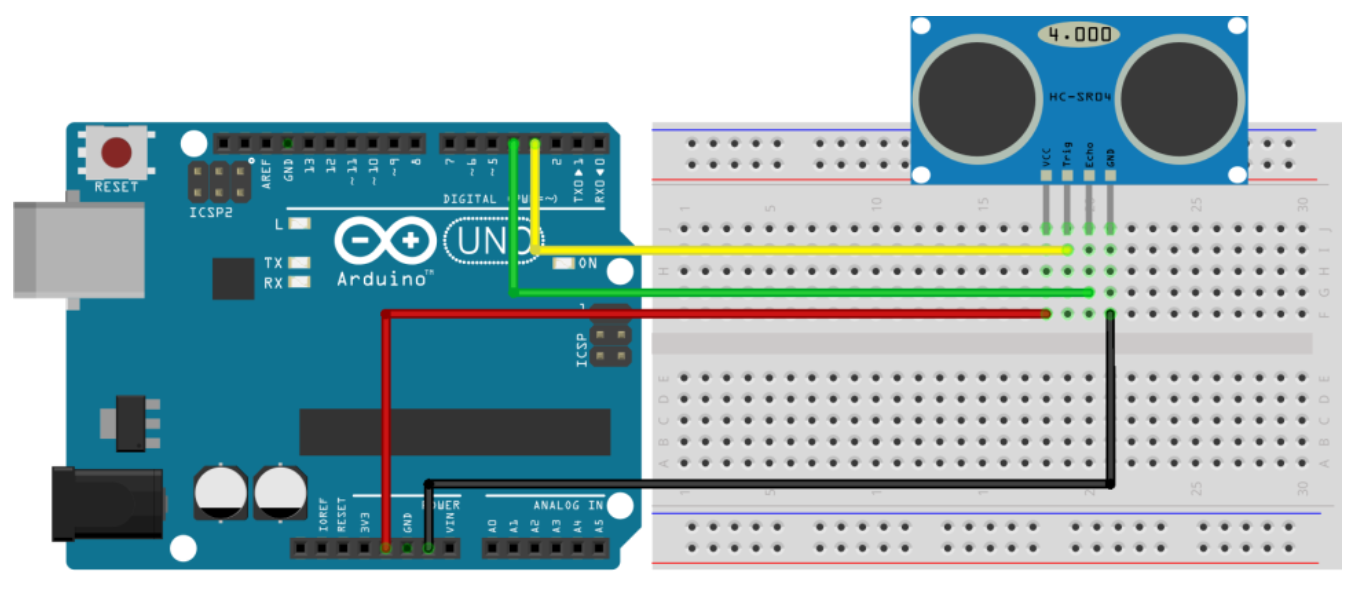
HC-SR04 | Arduino UNO |
GND | GND |
VCC | 5V |
Trig | D3(changeable) |
Echo | D4(changeable) |
Example Code
This example checks the distance on the serial monitor.
#define trigPin 3
#define echoPin 4
// Variable to hold the round-trip time of ultrasonic waves
double pulseTime;
void setup()
{
// Set the mode of each pin
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
// Initialize serial communication at 9600 baud rate
Serial.begin(9600);
delay(2000);
Serial.println("Connection successful");
delay(500);
// Initialize trig signal
digitalWrite(trigPin, LOW);
}
void loop()
{
// Start ultrasonic signal
digitalWrite(trigPin, HIGH);
// Generate ultrasonic waves for 10µs as per sensor specifications
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Measure round-trip time of ultrasonic waves
pulseTime = pulseIn(echoPin, HIGH);
// Calculate distance to the object
double distance_cm = pulseTime * 17 / 1000;
double distance_m = distance_cm / 100;
// Display error if the measurement is out of the sensor's specifications
if ((distance_cm < 400) && (distance_cm > 2))
{
Serial.print("Distance (cm) = ");
Serial.println(distance_cm);
Serial.print("Distance (m) = ");
Serial.println(distance_m);
Serial.println("--------------------------------------------------");
}
else
{
Serial.println("Out of sensor range");
}
delay(1000);
}