RFID,NFC Module(RC522)
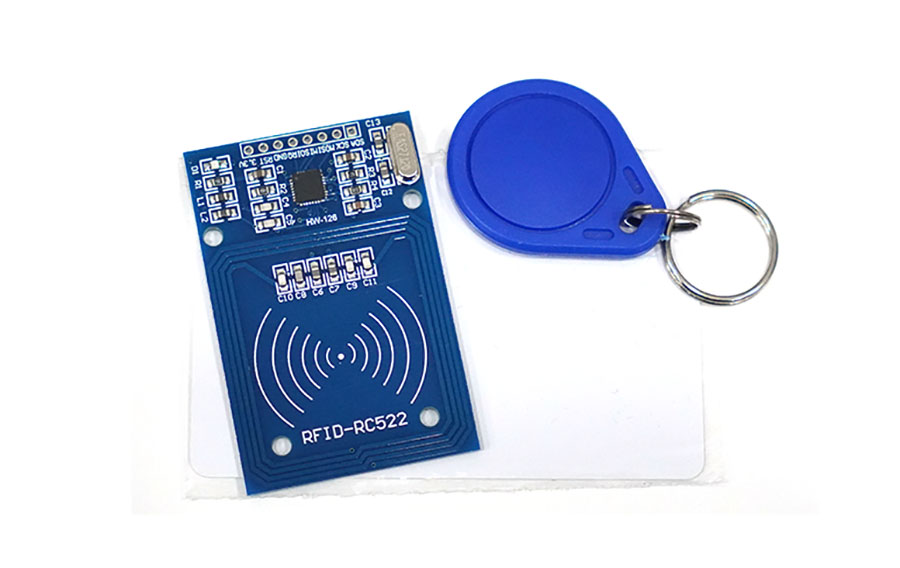
This is an RFID reader utilizing NFC communication method.
It can be used with Arduino and Raspberry Pi boards.
Specifications
- Operating Voltage: 3.3V
- Operating Current: 13mA ~ 26mA
- Interface: SPI
- Operating Frequency: 13.56MHz
Hardware
- Arduino
- RC522, NFC card/key
- Jumper cables
Connection
RC522 | Arduino | |||||
3.3V | 3.3V | |||||
RST | 9 | |||||
GND | GND | |||||
MISO | 12 | |||||
MOSI | 11 | |||||
SCK | 13 | |||||
SDA | 10 |
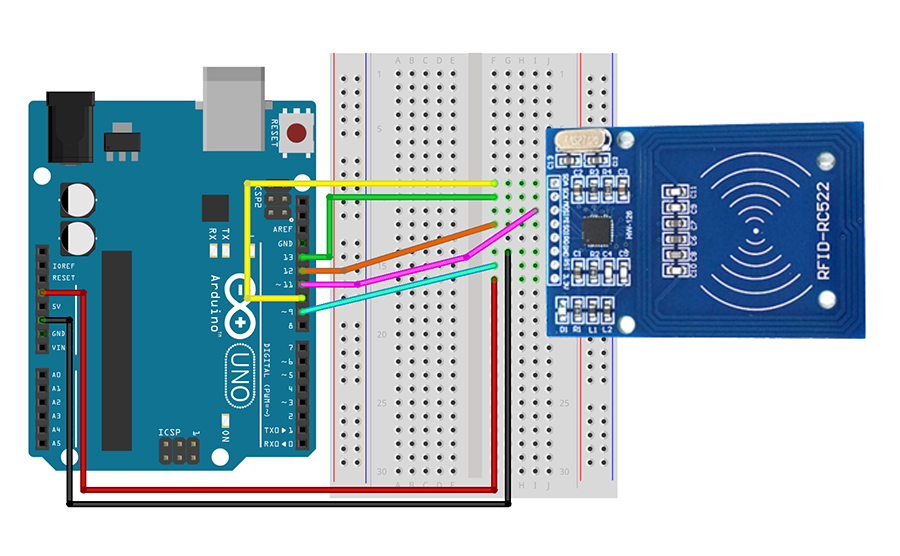
Libraries
- MFRC522 (by GithubCommunity)
- SPI (default included library)
Arduino Libraries
Example Code
Prints the tagged ID value to the serial monitor.
#include <SPI.h>
#include <MFRC522.h>
#define RST_PIN 9 // Reset pin is set to pin 9
#define SS_PIN 10 // SS pin is set to pin 10
// SS pin is the pin responsible for data exchange (SS = Slave Selector)
MFRC522 mfrc(SS_PIN, RST_PIN); // Creating the mfrc object to utilize the MFR522
void setup() {
Serial.begin(9600); // Serial communication, baud rate is set to 9600
SPI.begin(); // Initializing SPI
// (SPI: Communication method between one master and multiple slaves)
mfrc.PCD_Init(); // Initializing MFRC522
}
void loop() {
if (!mfrc.PICC_IsNewCardPresent() || !mfrc.PICC_ReadCardSerial()) {
// When no tag is detected or ID is not read
delay(500); // 0.5 second delay
return; // Return
}
Serial.print("Card UID:"); // Printing the tag's ID
for (byte i = 0; i < 4; i++) { // Loop to print the tag's ID, up to the tag's ID size (4)
Serial.print(mfrc.uid.uidByte[i]); // Printing from mfrc.uid.uidByte[0] to mfrc.uid.uidByte[3]
Serial.print(" "); // Printing space between IDs
}
Serial.println();
}
Execution Result
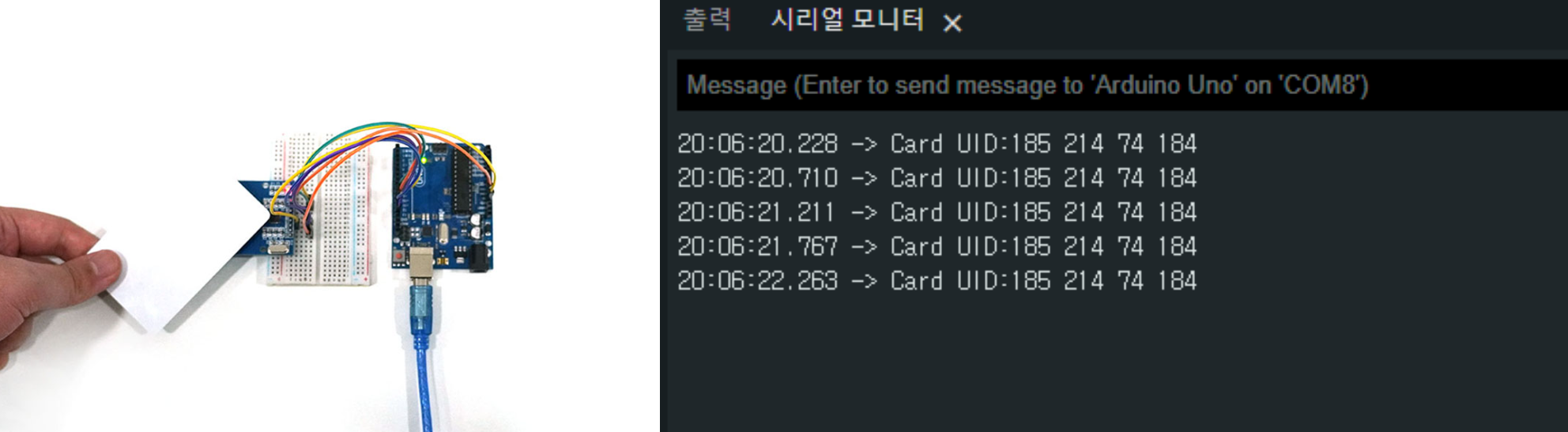