3-Color Traffic Light LED
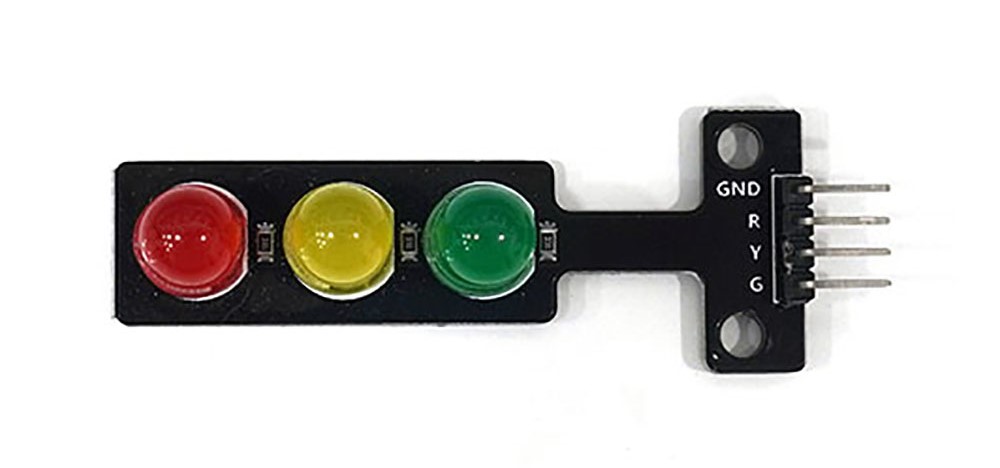
This is a module using three LEDs in the form of a traffic light: Red, Yellow, and Green.
Specifications
- Operating Voltage: 5V
- Operating Current: 20mA for each LED
Required Hardware
- 3-Color Traffic Light LED module
- Arduino UNO
- UNO Cable
- F-M Cable (4 pieces)
Connection
For the RYG terminals, you can set any pin number you want as OUTPUT to use.
3-Color Traffic Light LED | Arduino UNO |
---|---|
GND | GND |
R | D3 |
Y | D4 |
G | D5 |
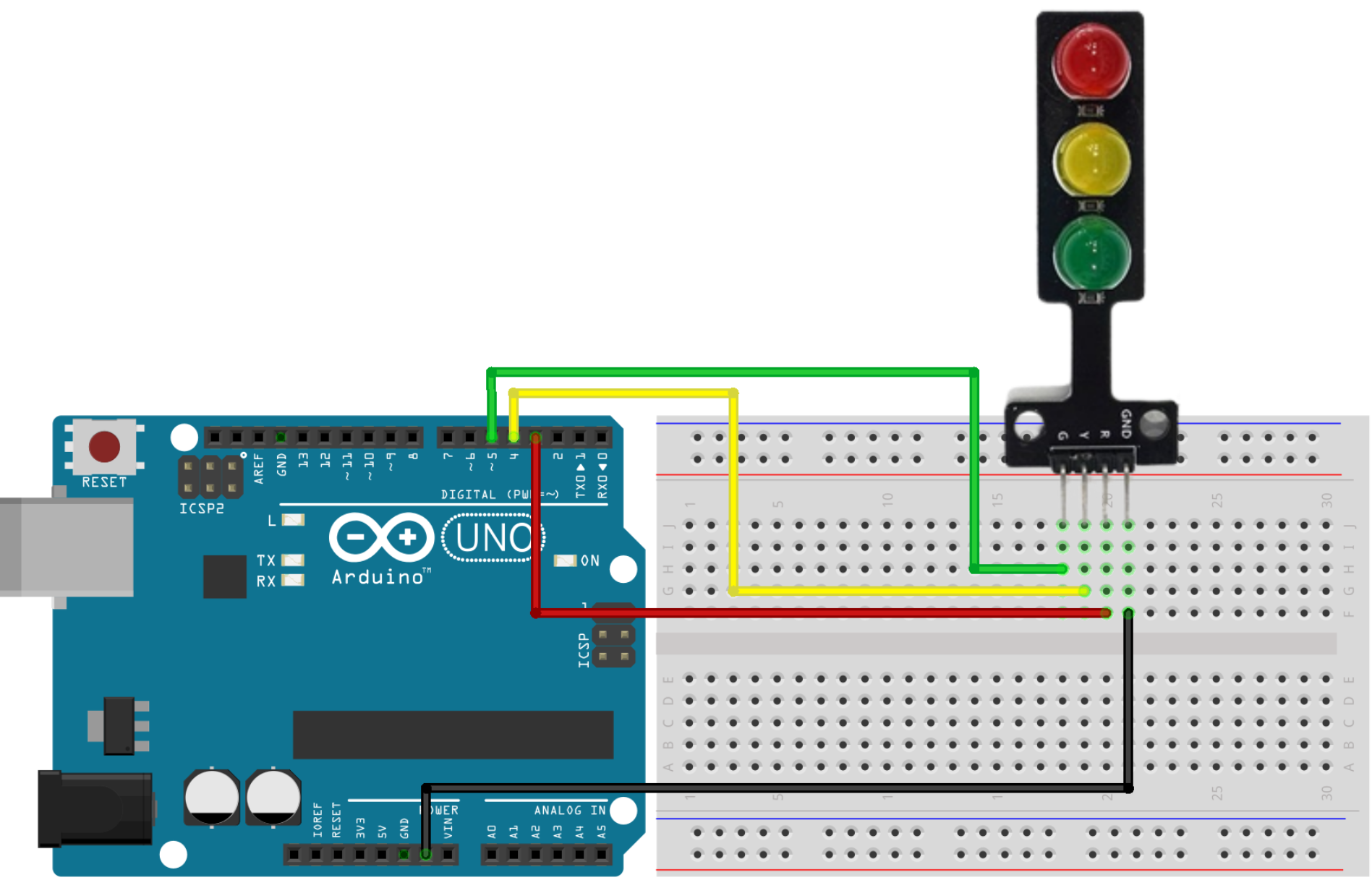
Example Code
// Define the pin numbers.
#define ledR 3
#define ledY 4
#define ledG 5
void setup()
{
// Set the pins to OUTPUT.
pinMode(ledR, OUTPUT);
pinMode(ledY, OUTPUT);
pinMode(ledG, OUTPUT);
}
void loop()
{
// Create the LED lighting loop.
// Lighting sequence (R -> off -> Y -> off -> G -> off -> All on -> All off)
turnON(3);
delay(500);
turnOFF(3);
delay(500);
turnON(4);
delay(500);
turnOFF(4);
delay(500);
turnON(5);
delay(500);
turnOFF(5);
delay(500);
turnON(1);
delay(500);
turnOFF(1);
delay(500);
}
// Function to turn the LED on.
void turnON(int x)
{
// Operate 3,4,5 for R,Y,G respectively, and 1 turns all LEDs on.
if (x == 3)
{
digitalWrite(ledR, HIGH);
}
else if (x == 4)
{
digitalWrite(ledY, HIGH);
}
else if (x == 5)
{
digitalWrite(ledG, HIGH);
}
else if (x == 1)
{
digitalWrite(ledR, HIGH);
digitalWrite(ledY, HIGH);
digitalWrite(ledG, HIGH);
}
}
// Function to turn the LED off.
void turnOFF(int x)
{
// Operate 3,4,5 for R,Y,G respectively, and 1 turns all LEDs off.
if (x == 3)
{
digitalWrite(ledR, LOW);
}
else if (x == 4)
{
digitalWrite(ledY, LOW);
}
else if (x == 5)
{
digitalWrite(ledG, LOW);
}
else if (x == 1)
{
digitalWrite(ledR, LOW);
digitalWrite(ledY, LOW);
digitalWrite(ledG, LOW);
}
}
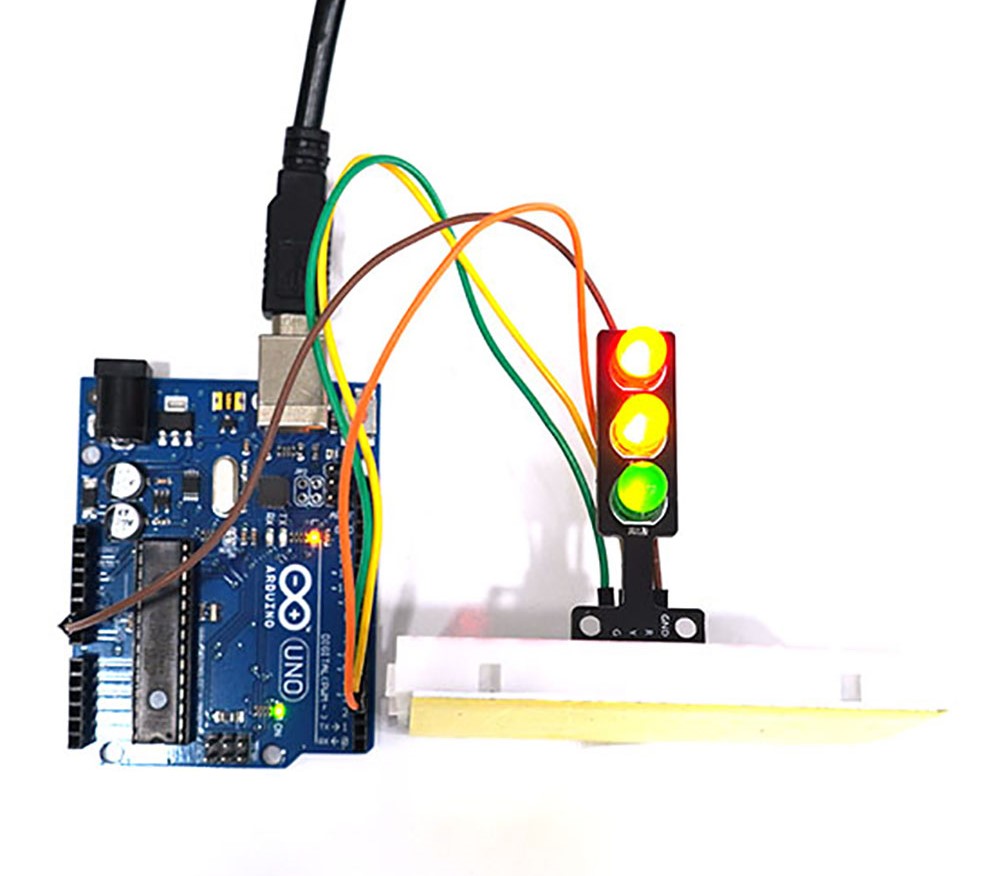