4 bits Segment Display(TM1637)
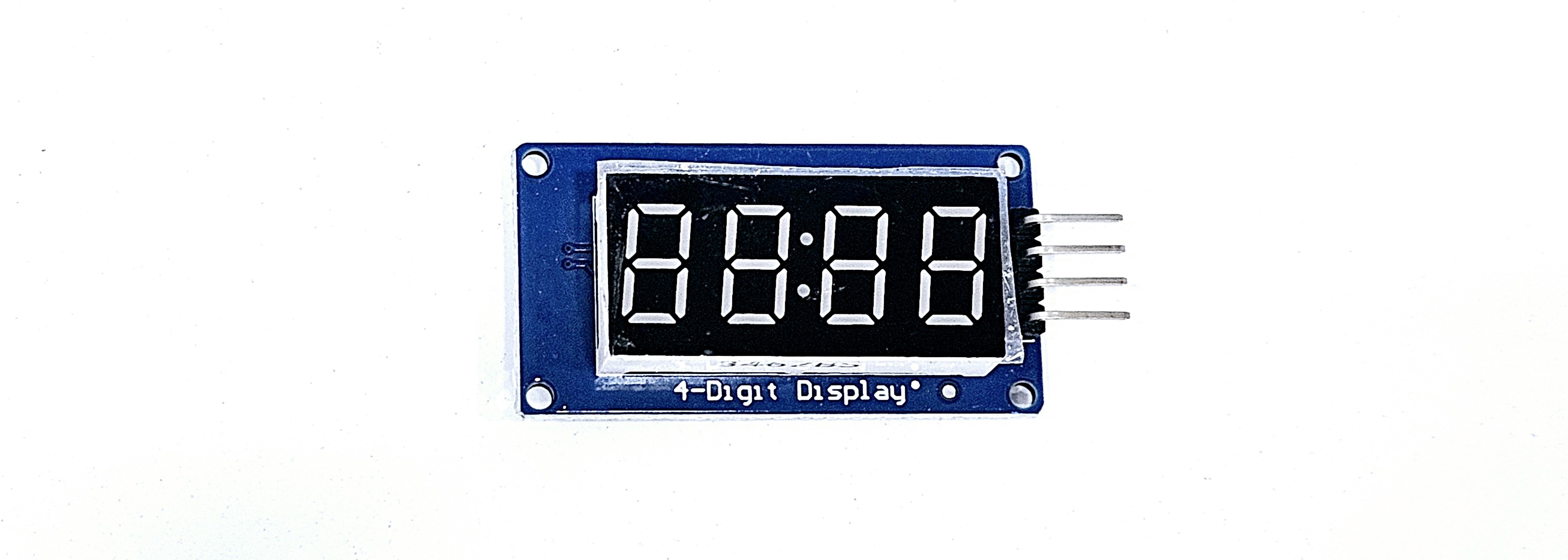
Overview
TM1637 is a driver IC for controlling 7-segment displays, which can be easily connected to microcontrollers like Arduino. It features a colon (:) display between the numbers, making it particularly popular for implementing digital clocks, and is widely used in various electronic projects such as thermometers and counters.
Specifications
TM1637 Module Specifications
List | Specifications |
---|---|
Power Supply | 3.3V ~ 5V |
Interface | I2C (TWI) |
Display | 7segment Display (4자리) |
Control Pin | CLK (Clock), DIO (Data) |
Temperature Range | -20°C ~ 70°C |
Size | 42 * 29 * 12 (mm) |
Mounting Hole
List | Details |
---|---|
Number of Holes | 4 M2 Screw Holes |
Hole Size | 2.2mm |
Usage Example
Circuit Configuration
Pin | Arduino |
---|---|
GND | GND |
VCC | 5V |
DIO | D3 |
CLK | D2 |
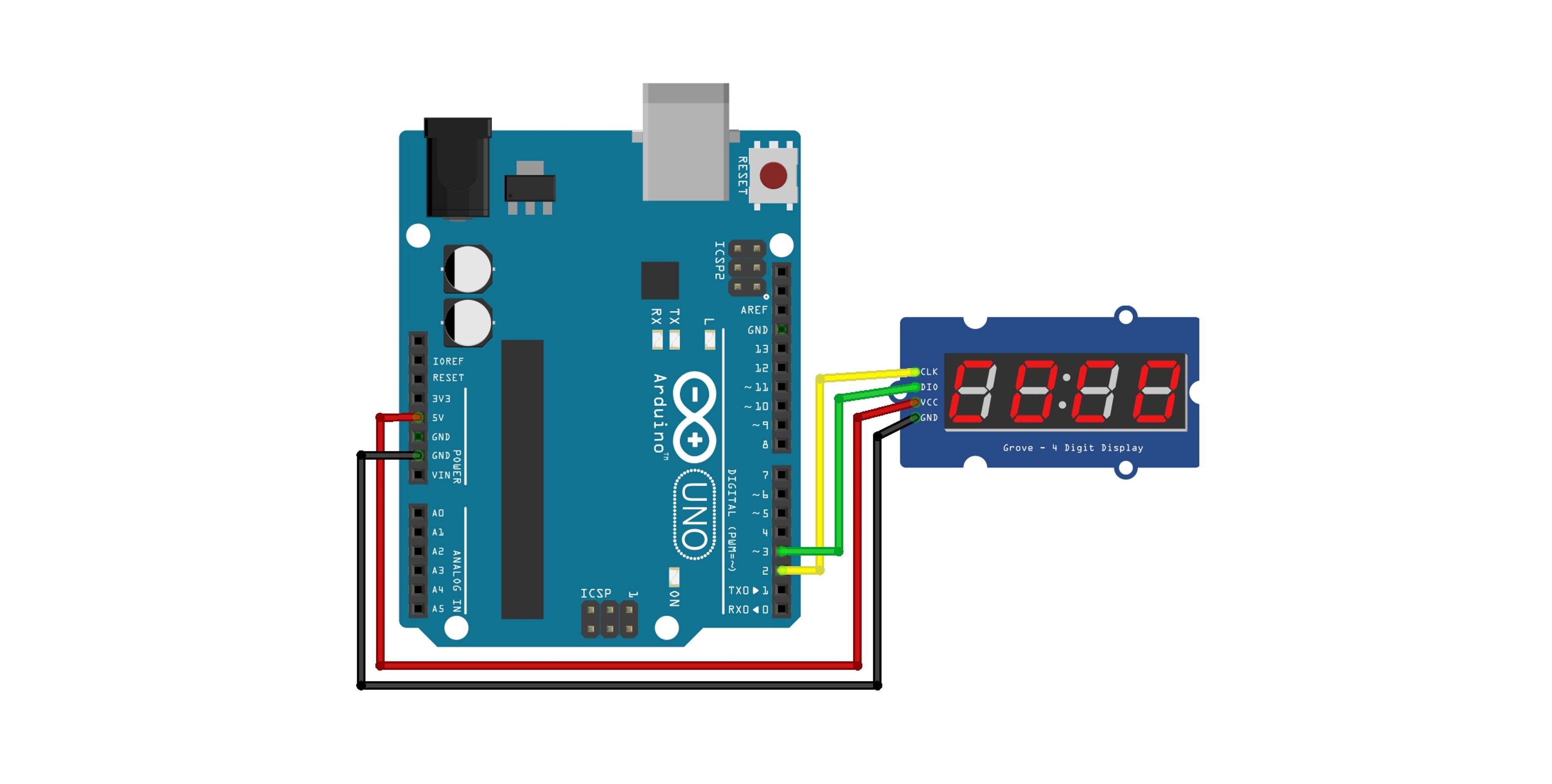
Library
TM1637Display.h library is used.
You can easily find and install TM1637 by searching in the library manager.
For more detailed information related to the library, please refer to the 아두이노 라이브러리 document.
TM1637 Key Functions of the Library
Function | Explanation |
---|---|
TM1637Display(CLK, DIO, delay)
|
TM1637 object constructor. Sets the CLK and DIO pins. |
setBrightness(uint8_t b, OnOff)
|
Sets the brightness of the display (0x00 ~ 0x0f). |
showNumberDec(int num, bool leading_zero)
|
Displays an integer in decimal. If leading_zero is true, adds a leading 0. |
showNumberHex(uint8_t num, bool leading_zero)
|
Displays a number in hexadecimal. |
clear()
|
Clears the display. |
setSegments(const uint8_t segments[], uint8_t size)
|
Displays custom segments. |
1. Number Display
Displays numbers from 0 to 10, changing at intervals of 0.5 seconds. The displayed number can be easily modified up to 4 digits.
showNumberDec(int num, bool leading_zero)
|
Displays an integer in decimal. If leading_zero is true, adds a leading 0. |
If you modify the leading_zero part of showNumberDec to true, it will display the number 1 in the format 0001.
#include <TM1637Display.h>
#define CLK 2 // Clock Pin
#define DIO 3 // Data Pin
TM1637Display display(CLK, DIO);
void setup() {
display.setBrightness(0x0f); // Sets the brightness.
}
void loop() {
for (int i = 0; i <= 10; i++) {
display.showNumberDec(i, false); // Display Number
delay(500); // Wait for 0.5 seconds.
}
}
Execution Result
2. Digital Clock Display
Displays hours and minutes in a 24-hour format.
The showNumberDecEx function is an extended version of the showNumberDec function, used to display a dot in the center.
#include <TM1637Display.h>
#define CLK 2
#define DIO 3
TM1637Display display(CLK, DIO);
void setup() {
display.setBrightness(0x0f);
}
void loop() {
int hour = 12;
int minute = 0;
for (int i = 0; i < 60; i++) {
display.showNumberDecEx(hour * 100 + minute, 0b11100000, true); // 시간 표시
delay(60000); // Wait for 1 Minute.
minute = (minute + 1) % 60; // Increase minute
if (minute == 0) hour = (hour + 1) % 24; // Increase hour
}
}
Execution Result
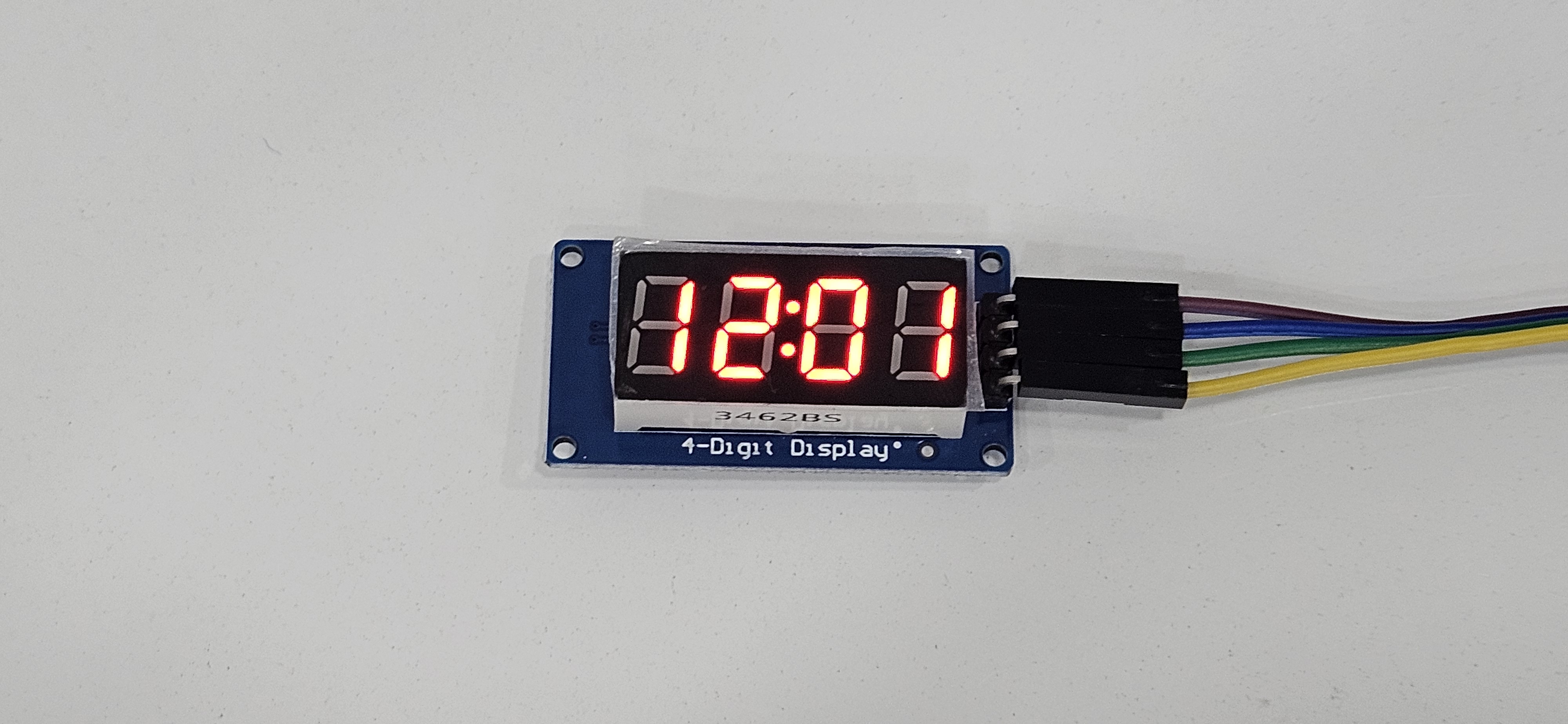
3. String Output Using SetSegments Function
The 7-segment patterns are organized as follows.
When the characters "GONG" are displayed on a 7-segment display, they appear as follows.
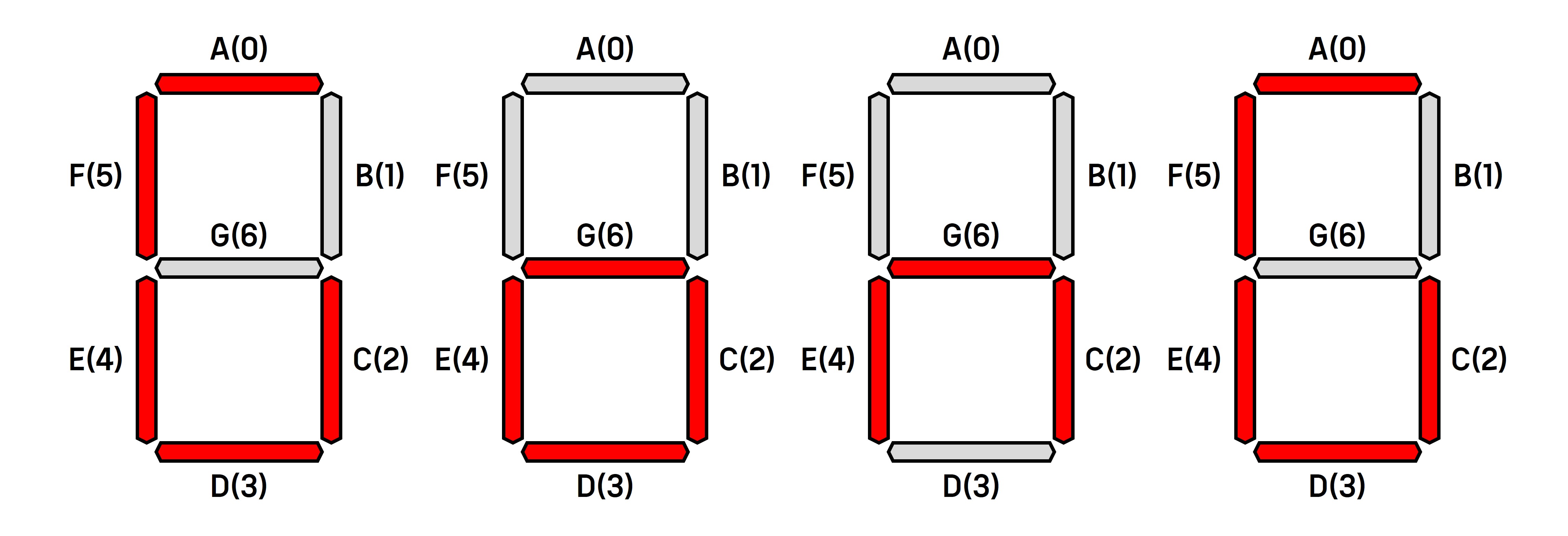
String | G | F | E | D | C | B | A | Binary | Hexadecimal |
---|---|---|---|---|---|---|---|---|---|
G | 0 | 1 | 1 | 1 | 1 | 0 | 1 | 111101 | 3d |
O | 1 | 0 | 1 | 1 | 1 | 0 | 0 | 1011100 | 5c |
N | 1 | 0 | 1 | 0 | 1 | 0 | 0 | 1010100 | 54 |
The parts that should be turned on are set to 1, and the parts that should be turned off are set to 0. After finding the binary representation for the desired character, you can express it in hexadecimal and apply it to the array.
The code implementing this is as follows.
#include <TM1637Display.h>
#define CLK 2
#define DIO 3
TM1637Display display(CLK, DIO);
byte data[] = {0x3d, 0x5c, 0x54, 0x3d};
void setup() {
display.setBrightness(0x0f); // Set maximum brightness
}
void loop() {
display.setSegments(data);
}
Execution Result
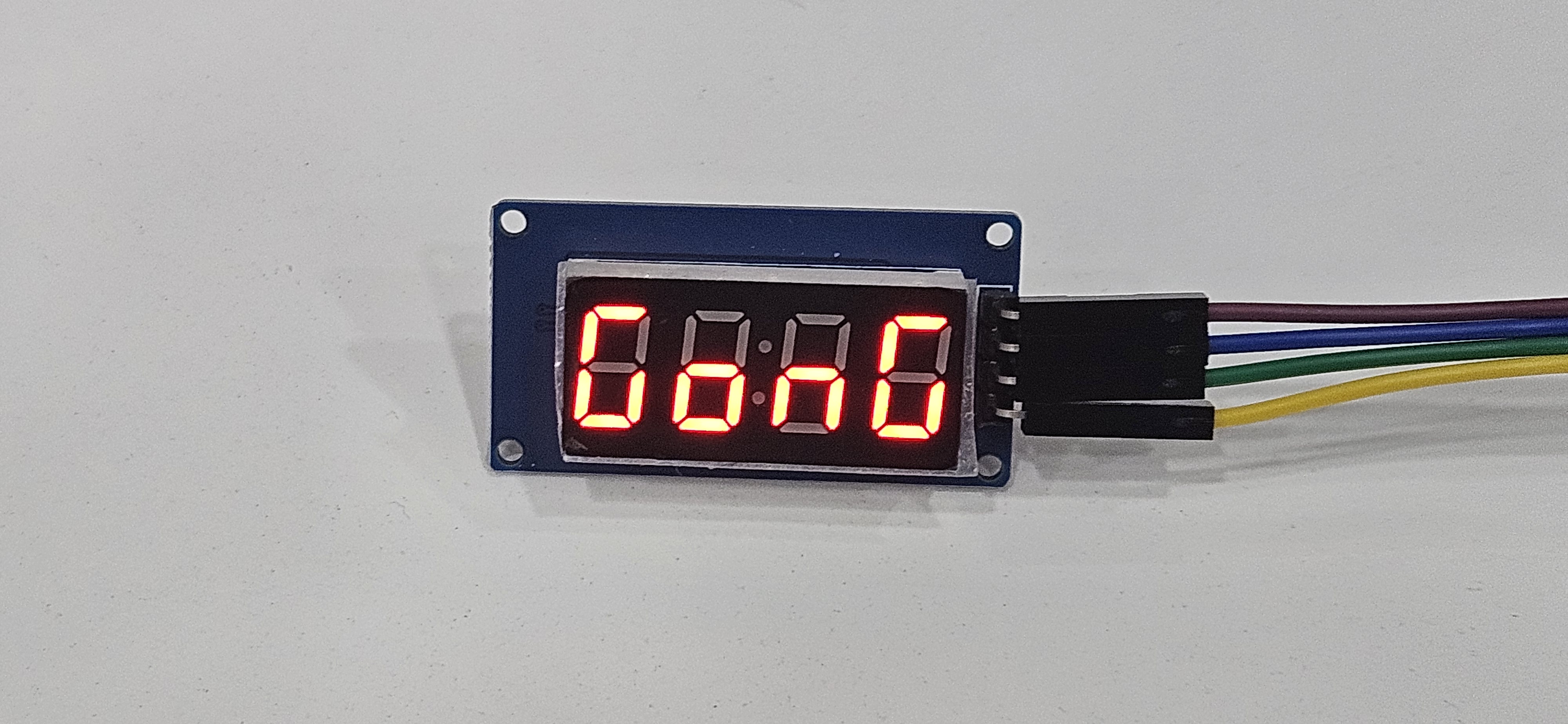
4. Scrolling Effect for Strings
This code displays characters longer than 4 characters by scrolling them to the left.
The characters "GONGZIPSA" are displayed as in the example above, scrolling one space to the left at a time.
#include <TM1637Display.h>
#define CLK 2
#define DIO 3
TM1637Display display(CLK, DIO);
// Given segment pattern
byte data[] = {0x3D, 0x5C, 0x54, 0x3D, 0x49, 0x30, 0x73, 0x6D, 0x77}; // Pattern for each character
void setup() {
display.setBrightness(0x0f); // Set maximum brightness
}
void loop() {
// Set string length and display size
int stringLength = sizeof(data) / sizeof(data[0]);
int displaySize = 4; // TM1637 can display up to 4 digits.
// Implementing a scrolling effect for strings.
for (int i = 0; i < stringLength + displaySize; i++) {
byte displayData[displaySize] = {0, 0, 0, 0}; // Initialize data to be displayed on the display.
// Set the character to be displayed based on the current position.
for (int j = 0; j < displaySize; j++) {
if (i + j >= 0 && i + j < stringLength) { // In the array range.
displayData[j] = data[i + j]; // Set the current character.
}
}
display.setSegments(displayData); // Display Segment
delay(500); // Wait for 0.5 seconds
}
}
Execution Result