Joystick Module(KY-023)
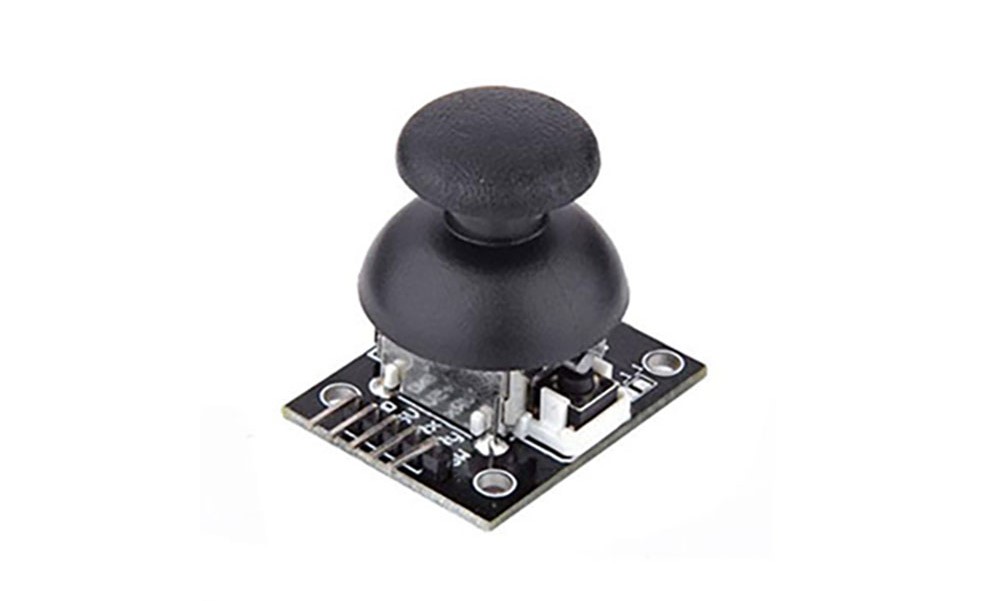
This module functions as a joystick, changing its x and y-axis values according to the resistance variations. Pressing the center button acts as a switch. It's commonly used in remote control cars, drones, and other applications requiring movement control.
Specifications
- Operating Voltage: 3.3V ~ 5V
- Analog output for x and y axes
- Digital output for the z-axis switch (without pull-up resistor)
Example Required Hardware
- Arduino board
- Jumper cables
- Joystick Module
- LCD 1602
Connection
Arduino | LCD | Joystick Module |
5V | VCC | 5V |
GND | GND | GND |
A1 | VRx | |
A0 | VRy | |
D2 | SW | |
A4 | SDA | |
A5 | SCL |
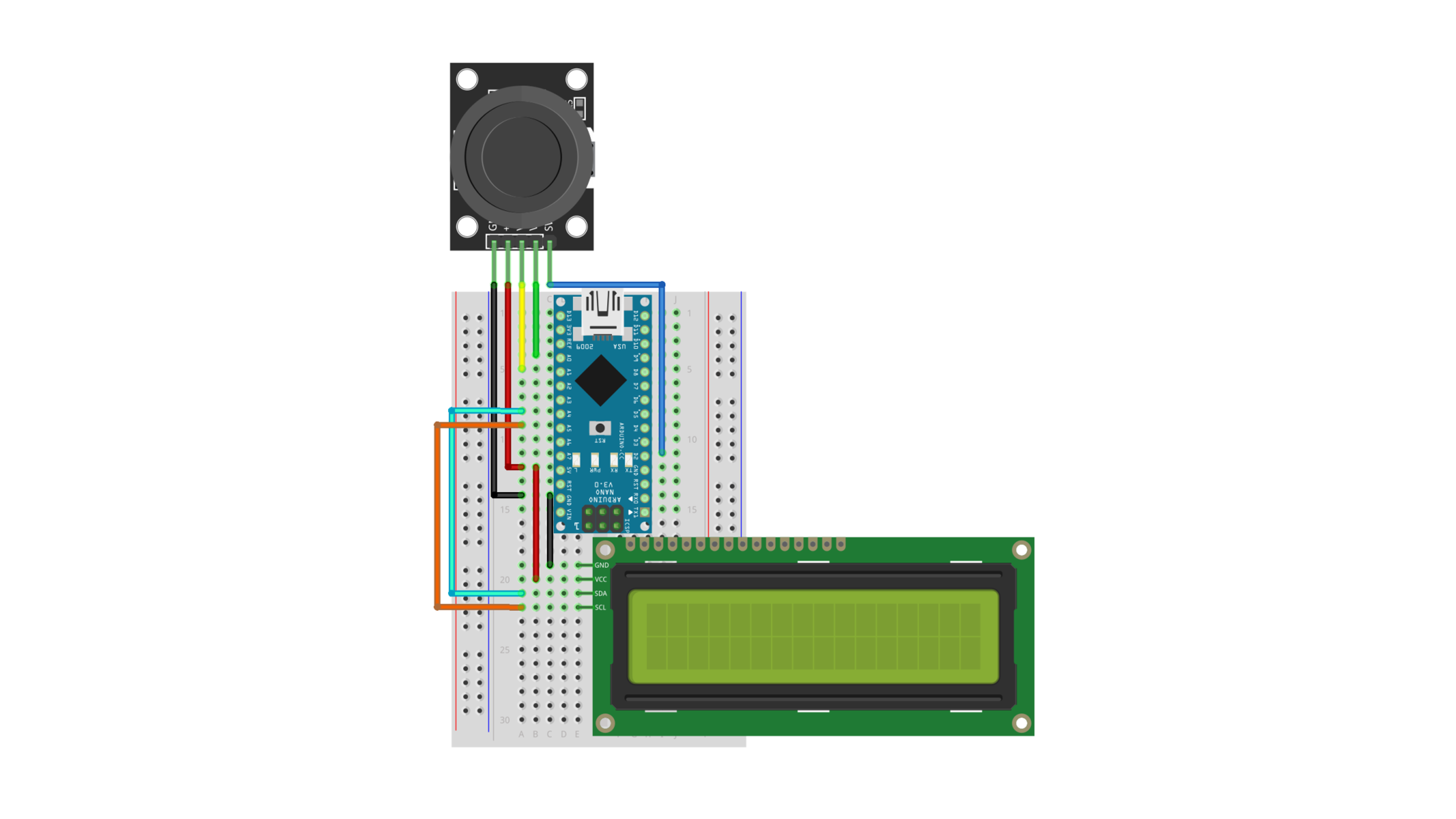
Example Code
This example displays the joystick's coordinates and switch state on an LCD screen.
Please refer to the link for instructions on using the LCD.
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27, 16, 2);
const int xAxis = A1;
const int yAxis = A0;
const int zSwitch = 2;
void setup() {
pinMode(zSwitch, INPUT_PULLUP);
lcd.init();
lcd.backlight();
lcd.setCursor(0, 0);
lcd.print("X:");
lcd.setCursor(8, 0);
lcd.print("Y:");
lcd.setCursor(0, 1);
lcd.print("Z : ");
}
void loop() {
for (int i = 2; i < 6; i++) {
lcd.setCursor(i, 0);
lcd.print(" ");
}
lcd.setCursor(2, 0);
lcd.print(analogRead(xAxis));
for (int i = 10; i < 14; i++) {
lcd.setCursor(i, 0);
lcd.print(" ");
}
lcd.setCursor(10, 0);
lcd.print(analogRead(yAxis));
lcd.setCursor(4, 1);
lcd.print(digitalRead(zSwitch));
delay(100);
}
Execution Result
For the operation video, please refer to the provided link.